When it comes to testing an e-learning site like ITLearn360, unit testing becomes the hero that ensures everything works as intended. Unit testing checks everything about a website, from how to log in to making sure the payment system works well. Let’s take a look at a practical and fun guide to unit testing with Selenium.
Why Unit Testing Matters
Think about the online learning platform you like the most. When you’re watching a tutorial on Java or taking a quiz, everything depends on small, functional components working together seamlessly. Unit testing ensures that these components work perfectly on their own, like logging in, searching for a course, or making payments.
Imagine making a cake: all the ingredients (flour, sugar, eggs) must be top-notch before being incorporated. It’s like tasting each ingredient to make sure it’s perfect before baking the whole cake.
Modules to Test in an E-learning Site
For ITLearn360, here are the must-test modules:
- User Management (Login, Registration, Password Reset)
- Course Catalog (Search, Filters, Course Details)
- Learning Modules (Videos, Quizzes)
- Payment Gateway (Successful and Failed Payments)
Let’s break them down with examples and pseudocode.
1. User Management: Keeping It Smooth
Example Scenario: Imagine entering your password on ITLearn360, and it doesn’t let you log in even with the correct credentials. Frustrating, right? That’s why we test!
Testing Login Functionality
@Test
public void testValidLogin() {
driver.get("https://www.itlearn360.com/login");
driver.findElement(By.id("username")).sendKeys("validUser");
driver.findElement(By.id("password")).sendKeys("validPassword");
driver.findElement(By.id("loginButton")).click();
Assert.assertEquals(driver.getCurrentUrl(), "https://www.itlearn360.com/dashboard");
}
What We’re Testing:
- Can users log in with correct credentials?
- Is the dashboard loaded after a successful login?
2. Course Catalog: Search and Filter Like a Pro
Example Scenario: Picture yourself searching for a Selenium course but getting cooking classes instead. Let’s fix that!
Testing Course Search
@Test
public void testCourseSearch() {
driver.get("https://www.itlearn360.com/courses");
driver.findElement(By.id("searchBar")).sendKeys("Selenium");
driver.findElement(By.id("searchButton")).click();
List<WebElement> courses = driver.findElements(By.className("courseTitle"));
for (WebElement course : courses) {
Assert.assertTrue(course.getText().contains("Selenium"));
}
}
What We’re Testing:
- Does the search return relevant courses?
Testing Filters
@Test
public void testCourseFilterByCategory() {
driver.get("https://www.itlearn360.com/courses");
driver.findElement(By.id("categoryFilter")).selectByVisibleText("Automation Testing");
List<WebElement> categories = driver.findElements(By.className("courseCategory"));
for (WebElement category : categories) {
Assert.assertEquals(category.getText(), "Automation Testing");
}
}
What We’re Testing:
- Do filters return courses only from the selected category?
3. Learning Modules: Videos and Quizzes That Work
Example Scenario: You’re halfway through a video tutorial, and it suddenly freezes. Nightmare! Let’s make sure it doesn’t happen.
Testing Video Streaming
@Test
public void testVideoStreaming() {
driver.get("https://www.itlearn360.com/course/java-module-1");
driver.findElement(By.id("playButton")).click();
WebElement videoPlayer = driver.findElement(By.id("videoPlayer"));
Assert.assertTrue(videoPlayer.getAttribute("class").contains("playing"));
}
What We’re Testing:
- Does the video player stream content seamlessly?
Testing Quiz Submission
@Test
public void testQuizSubmission() {
driver.get("https://www.itlearn360.com/quiz/java-basics");
driver.findElement(By.id("question1")).selectByValue("option1");
driver.findElement(By.id("question2")).selectByValue("option3");
driver.findElement(By.id("submitQuiz")).click();
WebElement score = driver.findElement(By.id("quizScore"));
Assert.assertTrue(score.isDisplayed());
}
What We’re Testing:
- Are quizzes calculated and displayed correctly?
4. Payment Gateway: Your Money, Safeguarded
Example Scenario: Imagine buying a course, but your payment fails without a reason. That’s a trust-breaker!
Testing Successful Payment
@Test
public void testSuccessfulPayment() {
driver.get("https://www.itlearn360.com/checkout");
driver.findElement(By.id("cardNumber")).sendKeys("4111111111111111");
driver.findElement(By.id("expiryDate")).sendKeys("12/25");
driver.findElement(By.id("cvv")).sendKeys("123");
driver.findElement(By.id("payNowButton")).click();
WebElement successMessage = driver.findElement(By.id("paymentSuccessMessage"));
Assert.assertTrue(successMessage.isDisplayed());
}
What We’re Testing:
- Are valid payments processed successfully?
Testing Failed Payment
@Test
public void testFailedPayment() {
driver.get("https://www.itlearn360.com/checkout");
driver.findElement(By.id("cardNumber")).sendKeys("1234567890123456");
driver.findElement(By.id("expiryDate")).sendKeys("01/22");
driver.findElement(By.id("cvv")).sendKeys("000");
driver.findElement(By.id("payNowButton")).click();
WebElement errorMessage = driver.findElement(By.id("paymentErrorMessage"));
Assert.assertTrue(errorMessage.isDisplayed());
}
What We’re Testing:
- Do users receive meaningful error messages for failed transactions?
Integration: End-to-End Testing
Example Scenario: Let’s test the entire flow: log in, search for a course, enroll, and pay.
@Test
public void testEndToEndEnrollmentFlow() {
driver.get("https://www.itlearn360.com/login");
driver.findElement(By.id("username")).sendKeys("testuser");
driver.findElement(By.id("password")).sendKeys("testpassword");
driver.findElement(By.id("loginButton")).click();
driver.findElement(By.id("searchBar")).sendKeys("Selenium");
driver.findElement(By.id("searchButton")).click();
driver.findElement(By.linkText("Master Selenium Course")).click();
driver.findElement(By.id("enrollButton")).click();
driver.findElement(By.id("payNowButton")).click();
WebElement paymentSuccess = driver.findElement(By.id("paymentSuccessMessage"));
Assert.assertTrue(paymentSuccess.isDisplayed());
}
Final Thoughts
Unit testing for an e-learning platform like ITLearn360 is more than just running scripts—it’s about ensuring a flawless experience for your customers. Whether it’s a seamless login, a well-filtered course catalog, or secure payments, unit testing has your back.
Unit Testing Tools and Best Practices
- Tools: Selenium (for automation), TestNG (for testing framework), and Mock frameworks for isolated tests.
- Best Practices:
- Write independent tests for each unit.
- Mock dependencies like databases or APIs for accurate testing.
- Maintain a repository of reusable test scripts.
Why Choose Infotek Solutions for Automation Testing Training
At Infotek Solutions, our comprehensive training program equips you with the skills needed to excel in Automation Testing with Selenium. Learn how to write robust unit tests, automate end-to-end workflows, and master QA tools used by industry leaders.
Selenium Certification Training
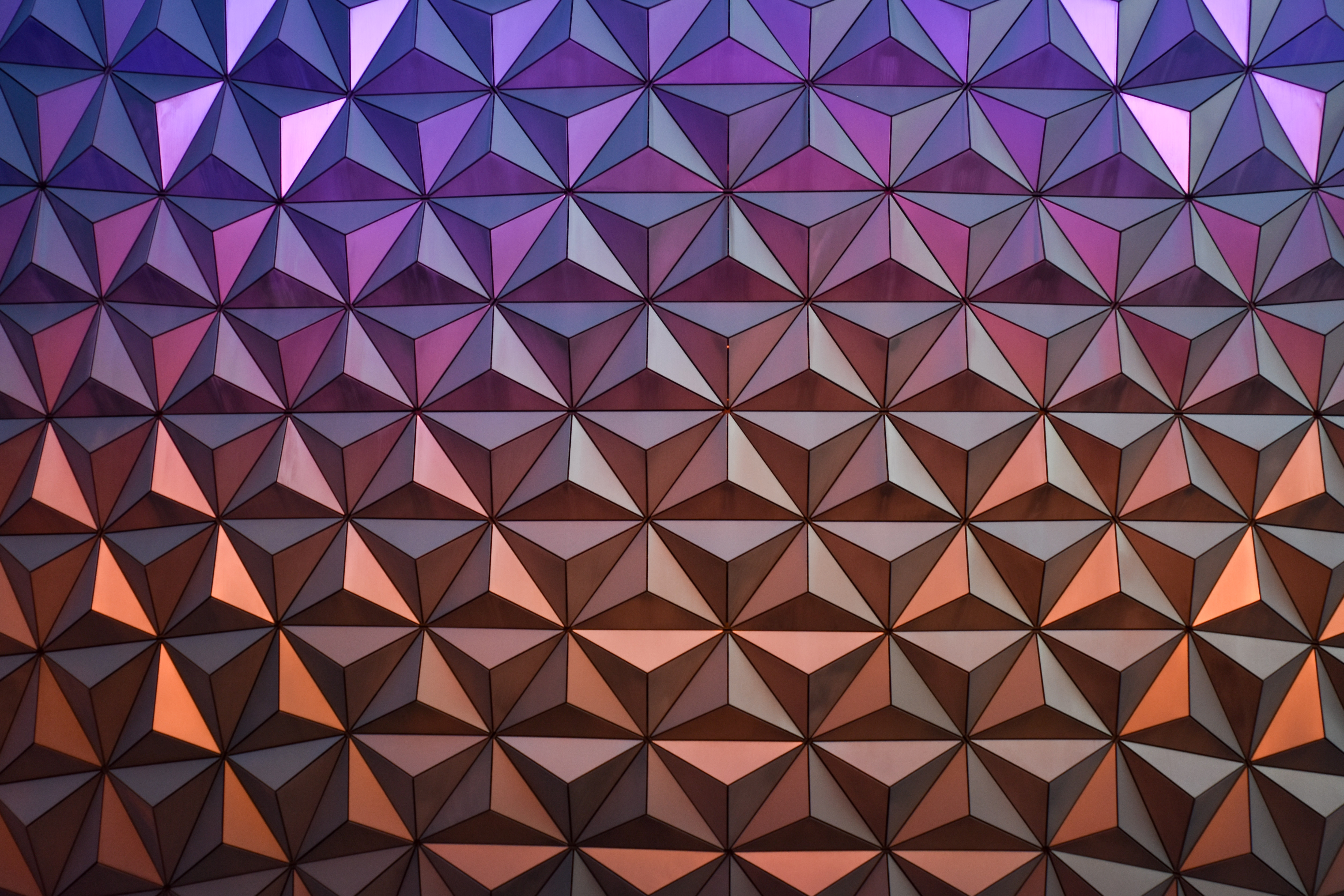
Ready to become a testing pro?
👉 Join Infotek Solutions’ Automation Testing Training Program to master Selenium, TestNG, and real-world testing techniques.